Find the Most Frequent Words in a Text Read From a File in Python
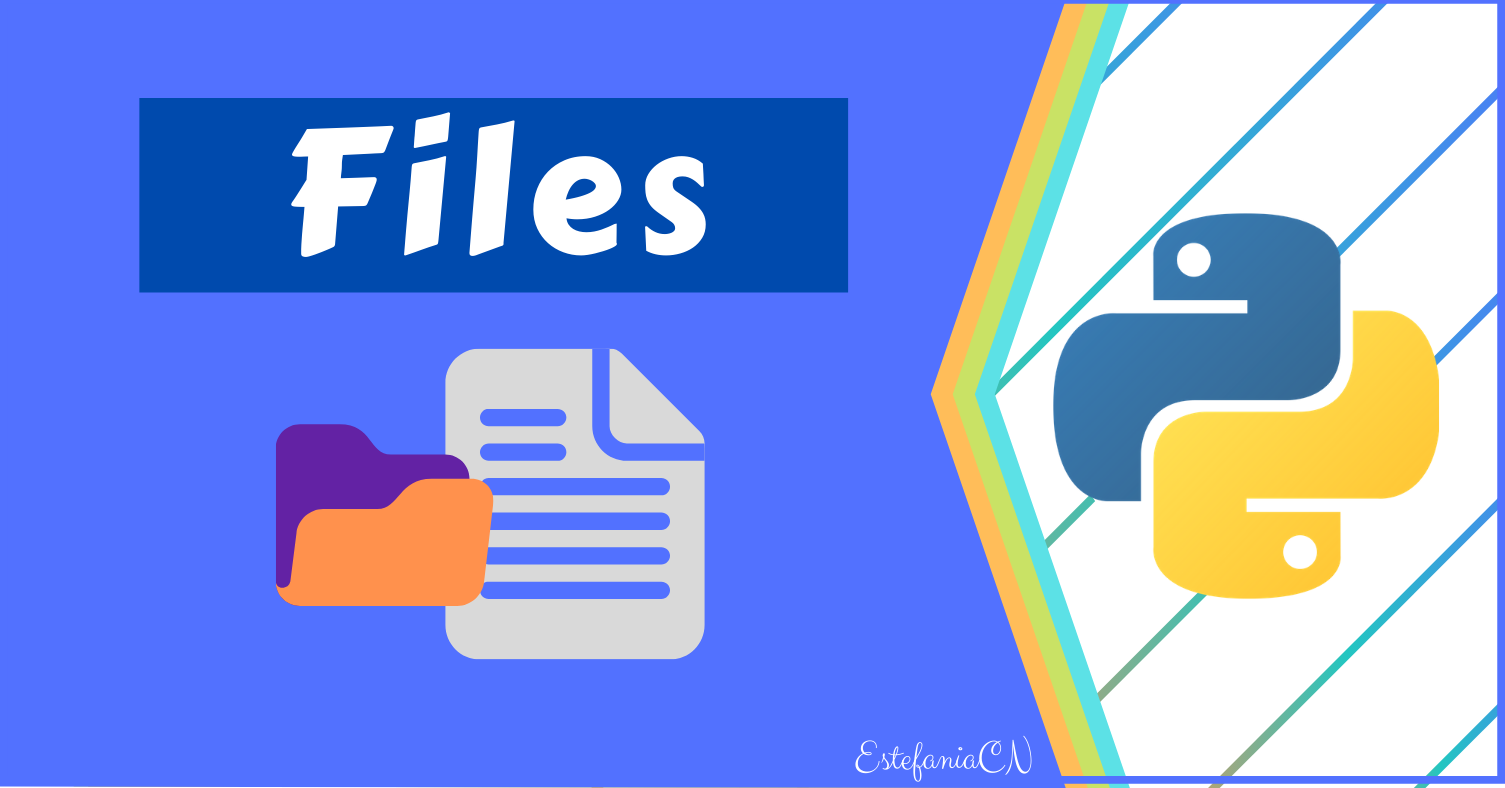
Welcome
Hi! If you lot want to learn how to work with files in Python, then this article is for you. Working with files is an important skill that every Python developer should acquire, so allow'due south get started.
In this commodity, you volition larn:
- How to open a file.
- How to read a file.
- How to create a file.
- How to alter a file.
- How to close a file.
- How to open up files for multiple operations.
- How to work with file object methods.
- How to delete files.
- How to work with context managers and why they are useful.
- How to handle exceptions that could exist raised when you piece of work with files.
- and more!
Let's begin! ✨
🔹 Working with Files: Basic Syntax
One of the most important functions that you lot will need to use equally you work with files in Python is open()
, a built-in function that opens a file and allows your program to apply it and work with it.
This is the basic syntax:
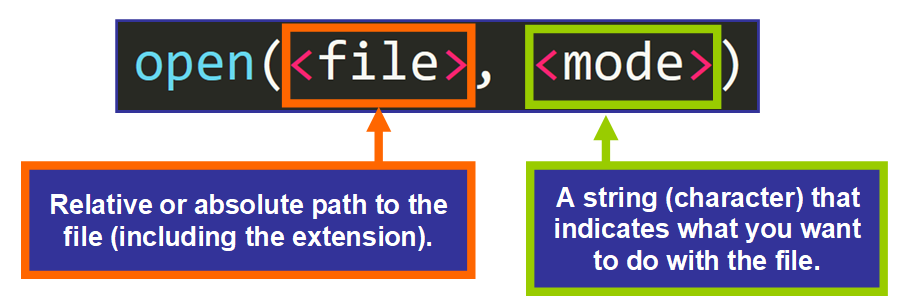
💡 Tip: These are the two well-nigh commonly used arguments to call this role. There are vi additional optional arguments. To learn more than nearly them, please read this article in the documentation.
First Parameter: File
The first parameter of the open up()
role is file
, the accented or relative path to the file that you are trying to work with.
We unremarkably utilize a relative path, which indicates where the file is located relative to the location of the script (Python file) that is calling the open()
role.
For example, the path in this function call:
open("names.txt") # The relative path is "names.txt"
Just contains the proper noun of the file. This can be used when the file that you are trying to open is in the same directory or folder as the Python script, like this:

Simply if the file is within a nested binder, similar this:

So we need to utilise a specific path to tell the role that the file is inside another folder.
In this example, this would be the path:
open("data/names.txt")
Notice that we are writing data/
first (the name of the folder followed past a /
) and then names.txt
(the proper noun of the file with the extension).
💡 Tip: The iii letters .txt
that follow the dot in names.txt
is the "extension" of the file, or its type. In this case, .txt
indicates that it'south a text file.
Second Parameter: Mode
The second parameter of the open()
part is the mode
, a string with i character. That single graphic symbol basically tells Python what you are planning to do with the file in your programme.
Modes available are:
- Read (
"r"
). - Suspend (
"a"
) - Write (
"w"
) - Create (
"10"
)
You can also choose to open up the file in:
- Text fashion (
"t"
) - Binary mode (
"b"
)
To use text or binary mode, you would need to add together these characters to the main mode. For example: "wb"
ways writing in binary fashion.
💡 Tip: The default modes are read ("r"
) and text ("t"
), which means "open for reading text" ("rt"
), so you don't need to specify them in open up()
if you want to apply them considering they are assigned by default. You lot tin simply write open(<file>)
.
Why Modes?
Information technology actually makes sense for Python to grant only certain permissions based what you are planning to do with the file, right? Why should Python allow your program to do more than necessary? This is basically why modes exist.
Retrieve virtually information technology — allowing a programme to do more than necessary can problematic. For example, if you only need to read the content of a file, it tin be dangerous to permit your programme to change information technology unexpectedly, which could potentially innovate bugs.
🔸 How to Read a File
At present that y'all know more than well-nigh the arguments that the open()
function takes, let's see how you tin can open a file and shop it in a variable to apply it in your program.
This is the basic syntax:

We are simply assigning the value returned to a variable. For example:
names_file = open("data/names.txt", "r")
I know you lot might be asking: what type of value is returned by open()
?
Well, a file object.
Let's talk a little bit well-nigh them.
File Objects
According to the Python Documentation, a file object is:
An object exposing a file-oriented API (with methods such as read() or write()) to an underlying resource.
This is basically telling us that a file object is an object that lets usa work and interact with existing files in our Python programme.
File objects take attributes, such equally:
- name: the name of the file.
- closed:
True
if the file is closed.False
otherwise. - mode: the fashion used to open the file.
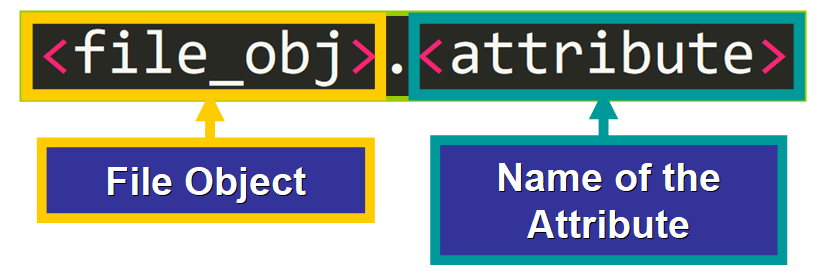
For example:
f = open("data/names.txt", "a") print(f.mode) # Output: "a"
Now let'due south see how yous can admission the content of a file through a file object.
Methods to Read a File
For us to be able to piece of work file objects, nosotros need to have a style to "collaborate" with them in our plan and that is exactly what methods practice. Let's come across some of them.
Read()
The first method that you need to learn about is read()
, which returns the entire content of the file as a cord.

Here we have an example:
f = open up("data/names.txt") impress(f.read())
The output is:
Nora Gino Timmy William
You can use the blazon()
function to confirm that the value returned by f.read()
is a string:
print(type(f.read())) # Output <class 'str'>
Yes, it'due south a string!
In this case, the entire file was printed because we did not specify a maximum number of bytes, but we can do this too.
Here we have an instance:
f = open up("data/names.txt") print(f.read(three))
The value returned is limited to this number of bytes:
Nor
❗️Important: You lot need to shut a file after the task has been completed to free the resources associated to the file. To exercise this, you need to call the close()
method, similar this:

Readline() vs. Readlines()
You can read a file line by line with these 2 methods. They are slightly different, then let's see them in item.
readline()
reads ane line of the file until it reaches the finish of that line. A trailing newline character (\n
) is kept in the string.
💡 Tip: Optionally, you can laissez passer the size, the maximum number of characters that you lot want to include in the resulting string.
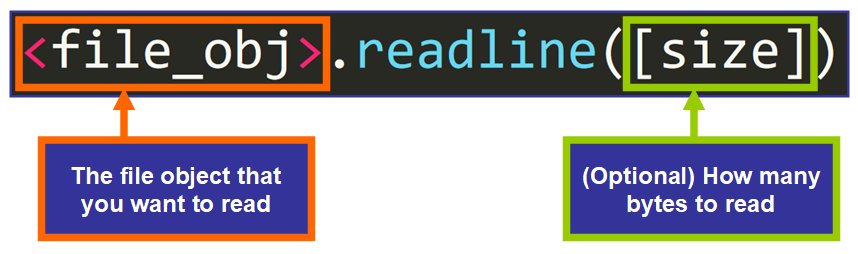
For example:
f = open up("data/names.txt") print(f.readline()) f.close()
The output is:
Nora
This is the starting time line of the file.
In dissimilarity, readlines()
returns a list with all the lines of the file as individual elements (strings). This is the syntax:

For instance:
f = open("data/names.txt") impress(f.readlines()) f.close()
The output is:
['Nora\n', 'Gino\n', 'Timmy\northward', 'William']
Notice that there is a \n
(newline character) at the cease of each string, except the last ane.
💡 Tip: You tin go the same listing with list(f)
.
You lot can work with this list in your programme by assigning information technology to a variable or using it in a loop:
f = open up("data/names.txt") for line in f.readlines(): # Do something with each line f.close()
We tin can also iterate over f
straight (the file object) in a loop:
f = open("information/names.txt", "r") for line in f: # Do something with each line f.shut()
Those are the chief methods used to read file objects. Now let'due south encounter how yous tin can create files.
🔹 How to Create a File
If you need to create a file "dynamically" using Python, you lot can do it with the "x"
mode.
Let's see how. This is the bones syntax:

Here's an example. This is my electric current working directory:

If I run this line of code:
f = open up("new_file.txt", "10")
A new file with that name is created:

With this manner, you can create a file and so write to it dynamically using methods that y'all will learn in but a few moments.
💡 Tip: The file will be initially empty until you change it.
A curious affair is that if y'all try to run this line again and a file with that name already exists, you volition see this mistake:
Traceback (most contempo call last): File "<path>", line 8, in <module> f = open up("new_file.txt", "x") FileExistsError: [Errno 17] File exists: 'new_file.txt'
According to the Python Documentation, this exception (runtime error) is:
Raised when trying to create a file or directory which already exists.
Now that you know how to create a file, let'south run into how y'all tin change information technology.
🔸 How to Alter a File
To modify (write to) a file, you need to use the write()
method. You lot have two ways to do information technology (suspend or write) based on the mode that you cull to open it with. Let'southward see them in detail.
Append
"Appending" means adding something to the end of some other thing. The "a"
fashion allows you to open a file to append some content to it.
For instance, if we take this file:
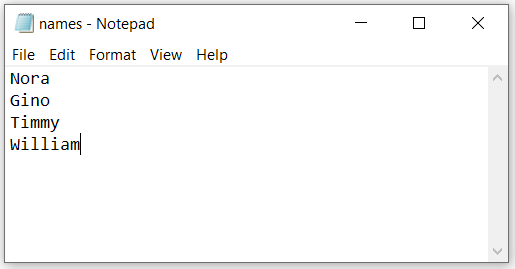
And nosotros want to add a new line to it, we can open it using the "a"
mode (append) and and then, call the write()
method, passing the content that nosotros want to append as argument.
This is the basic syntax to call the write()
method:

Here's an example:
f = open("data/names.txt", "a") f.write("\nNew Line") f.shut()
💡 Tip: Discover that I'm calculation \n
before the line to indicate that I want the new line to appear as a carve up line, not as a continuation of the existing line.
This is the file now, later on running the script:

💡 Tip: The new line might not be displayed in the file until f.close()
runs.
Write
Sometimes, you may want to delete the content of a file and supercede it entirely with new content. Y'all tin do this with the write()
method if yous open up the file with the "due west"
manner.
Here we have this text file:
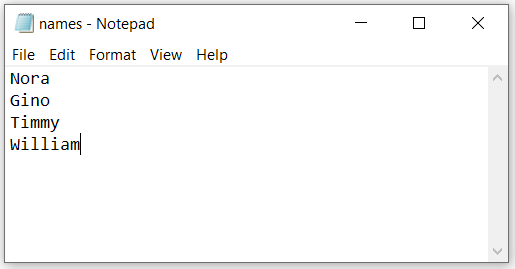
If I run this script:
f = open("data/names.txt", "west") f.write("New Content") f.close()
This is the result:
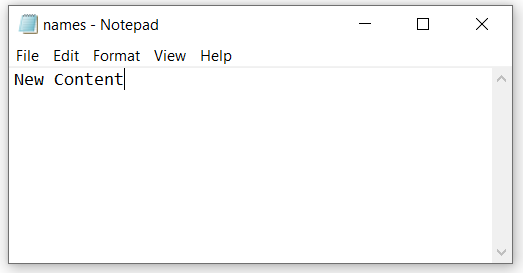
Equally you can meet, opening a file with the "due west"
way and and so writing to information technology replaces the existing content.
💡 Tip: The write()
method returns the number of characters written.
If you want to write several lines at one time, you lot can use the writelines()
method, which takes a list of strings. Each string represents a line to be added to the file.
Here's an instance. This is the initial file:
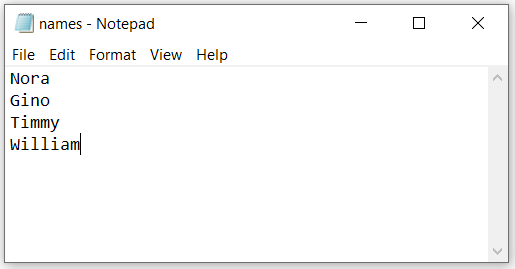
If we run this script:
f = open("data/names.txt", "a") f.writelines(["\nline1", "\nline2", "\nline3"]) f.close()
The lines are added to the cease of the file:
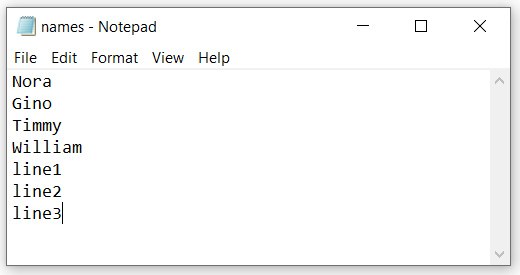
Open File For Multiple Operations
At present y'all know how to create, read, and write to a file, but what if y'all want to exercise more than one affair in the same program? Allow'due south see what happens if we attempt to practise this with the modes that you have learned and then far:
If y'all open a file in "r"
manner (read), and then endeavor to write to it:
f = open("information/names.txt") f.write("New Content") # Trying to write f.close()
You will get this error:
Traceback (most contempo call last): File "<path>", line 9, in <module> f.write("New Content") io.UnsupportedOperation: not writable
Similarly, if y'all open up a file in "w"
mode (write), then try to read it:
f = open("data/names.txt", "w") print(f.readlines()) # Trying to read f.write("New Content") f.shut()
You will meet this mistake:
Traceback (most recent phone call last): File "<path>", line 14, in <module> print(f.readlines()) io.UnsupportedOperation: not readable
The aforementioned will occur with the "a"
(append) mode.
How tin we solve this? To exist able to read a file and perform another performance in the same program, y'all demand to add the "+"
symbol to the fashion, like this:
f = open("information/names.txt", "w+") # Read + Write
f = open("data/names.txt", "a+") # Read + Append
f = open("data/names.txt", "r+") # Read + Write
Very useful, correct? This is probably what y'all will apply in your programs, merely exist sure to include only the modes that you lot need to avoid potential bugs.
Sometimes files are no longer needed. Let's see how you can delete files using Python.
🔹 How to Delete Files
To remove a file using Python, you need to import a module called os
which contains functions that interact with your operating system.
💡 Tip: A module is a Python file with related variables, functions, and classes.
Particularly, you demand the remove()
function. This function takes the path to the file every bit argument and deletes the file automatically.

Let's come across an example. We want to remove the file called sample_file.txt
.

To do it, we write this code:
import os bone.remove("sample_file.txt")
- The first line:
import os
is called an "import argument". This statement is written at the top of your file and it gives you access to the functions defined in thebone
module. - The 2d line:
os.remove("sample_file.txt")
removes the file specified.
💡 Tip: you lot tin use an absolute or a relative path.
Now that you know how to delete files, let'due south see an interesting tool... Context Managers!
🔸 Come across Context Managers
Context Managers are Python constructs that will make your life much easier. By using them, you don't need to remember to shut a file at the terminate of your programme and you have access to the file in the particular part of the program that you choose.
Syntax
This is an example of a context director used to work with files:

💡 Tip: The trunk of the context director has to be indented, just like we indent loops, functions, and classes. If the code is not indented, it will not exist considered function of the context manager.
When the body of the context manager has been completed, the file closes automatically.
with open("<path>", "<mode>") as <var>: # Working with the file... # The file is closed here!
Case
Here's an example:
with open("information/names.txt", "r+") equally f: print(f.readlines())
This context managing director opens the names.txt
file for read/write operations and assigns that file object to the variable f
. This variable is used in the body of the context manager to refer to the file object.
Trying to Read it Over again
After the body has been completed, the file is automatically airtight, so it can't be read without opening it again. Only wait! Nosotros have a line that tries to read it again, right hither below:
with open("data/names.txt", "r+") as f: impress(f.readlines()) print(f.readlines()) # Trying to read the file again, outside of the context manager
Let's see what happens:
Traceback (most recent phone call last): File "<path>", line 21, in <module> print(f.readlines()) ValueError: I/O operation on airtight file.
This error is thrown because nosotros are trying to read a closed file. Awesome, right? The context managing director does all the heavy work for united states, it is readable, and curtailed.
🔹 How to Handle Exceptions When Working With Files
When you're working with files, errors can occur. Sometimes yous may not have the necessary permissions to modify or access a file, or a file might not fifty-fifty exist.
As a programmer, you need to foresee these circumstances and handle them in your program to avoid sudden crashes that could definitely affect the user feel.
Allow's see some of the virtually common exceptions (runtime errors) that you might notice when you lot work with files:
FileNotFoundError
According to the Python Documentation, this exception is:
Raised when a file or directory is requested only doesn't exist.
For example, if the file that you're trying to open doesn't be in your current working directory:
f = open("names.txt")
You will see this error:
Traceback (most contempo phone call last): File "<path>", line eight, in <module> f = open("names.txt") FileNotFoundError: [Errno 2] No such file or directory: 'names.txt'
Allow's break this error down this line by line:
-
File "<path>", line 8, in <module>
. This line tells you that the error was raised when the code on the file located in<path>
was running. Specifically, whenline viii
was executed in<module>
. -
f = open("names.txt")
. This is the line that caused the error. -
FileNotFoundError: [Errno 2] No such file or directory: 'names.txt'
. This line says that aFileNotFoundError
exception was raised because the file or directorynames.txt
doesn't exist.
💡 Tip: Python is very descriptive with the error messages, right? This is a huge advantage during the process of debugging.
PermissionError
This is some other common exception when working with files. According to the Python Documentation, this exception is:
Raised when trying to run an operation without the acceptable access rights - for instance filesystem permissions.
This exception is raised when you lot are trying to read or modify a file that don't have permission to admission. If you try to do and then, you will run across this fault:
Traceback (most recent call terminal): File "<path>", line viii, in <module> f = open("<file_path>") PermissionError: [Errno 13] Permission denied: 'data'
IsADirectoryError
Co-ordinate to the Python Documentation, this exception is:
Raised when a file operation is requested on a directory.
This particular exception is raised when you endeavor to open or piece of work on a directory instead of a file, so be really careful with the path that yous pass every bit argument.
How to Handle Exceptions
To handle these exceptions, yous tin employ a try/except statement. With this statement, y'all can "tell" your program what to do in case something unexpected happens.
This is the bones syntax:
attempt: # Try to run this lawmaking except <type_of_exception>: # If an exception of this type is raised, finish the procedure and bound to this cake
Here you tin can see an case with FileNotFoundError
:
try: f = open("names.txt") except FileNotFoundError: print("The file doesn't exist")
This basically says:
- Try to open the file
names.txt
. - If a
FileNotFoundError
is thrown, don't crash! Simply print a descriptive statement for the user.
💡 Tip: Yous tin can cull how to handle the situation by writing the appropriate code in the except
block. Perhaps you could create a new file if it doesn't be already.
To close the file automatically after the task (regardless of whether an exception was raised or not in the try
block) you can add together the finally
block.
endeavor: # Endeavor to run this code except <exception>: # If this exception is raised, stop the process immediately and spring to this cake finally: # Practice this later running the code, even if an exception was raised
This is an instance:
try: f = open("names.txt") except FileNotFoundError: print("The file doesn't exist") finally: f.close()
There are many ways to customize the try/except/finally statement and you tin can even add together an else
cake to run a block of code merely if no exceptions were raised in the try
block.
💡 Tip: To learn more about exception treatment in Python, yous may similar to read my article: "How to Handle Exceptions in Python: A Detailed Visual Introduction".
🔸 In Summary
- You can create, read, write, and delete files using Python.
- File objects have their own fix of methods that you tin use to work with them in your program.
- Context Managers help yous piece of work with files and manage them past endmost them automatically when a task has been completed.
- Exception handling is key in Python. Mutual exceptions when you are working with files include
FileNotFoundError
,PermissionError
andIsADirectoryError
. They can exist handled using effort/except/else/finally.
I actually hope you lot liked my article and found it helpful. Now yous can work with files in your Python projects. Check out my online courses. Follow me on Twitter. ⭐️
Larn to lawmaking for free. freeCodeCamp's open source curriculum has helped more 40,000 people become jobs as developers. Get started
Source: https://www.freecodecamp.org/news/python-write-to-file-open-read-append-and-other-file-handling-functions-explained/
0 Response to "Find the Most Frequent Words in a Text Read From a File in Python"
Post a Comment